I have list of users. When I click submit detail then
I want to create PDF file dynamically according to users detail and send with email to all users in nodejs.
This isn't really something that MailGun is designed to handle. You're responsible for creating the content and they're responsible for sending the content.
If you need to create a PDF dynamically, I'd recommend using Node or PHP to create your content as HTML/CSS and then using a library like html2pdf or a service like PDFShift to render your output in PDF form.
If you want to create a PDF dynamically i would suggest you to use pdf-creator-node
and for sending mails i would suggest you to use nodemailer
you can use this repo.
Check out Docamatic. It allows you to to create and send a PDF in a single API request.
{
"source": "https://my-site.com/invoice.html",
"email": {
"to": "accounts#my-customer.com",
"subject": "July Invoice"
}
}
Related
Recently we are building a application which send message through Microsoft BotFramework API
We are using the API as below
https://learn.microsoft.com/en-us/azure/bot-service/rest-api/bot-framework-rest-connector-api-reference?view=azure-bot-service-4.0#send-to-conversation
The following API allow us to send text or attachments by passing activities as parameters.
https://learn.microsoft.com/en-us/azure/bot-service/rest-api/bot-framework-rest-connector-api-reference?view=azure-bot-service-4.0#activity-object
Everything works well, and on our client side, text and attachments can be received normally as below.
However, recently we have to send rich text which contains text and image info. For example, admin user want to send text and pasted image together to client user.
So we are considering sending text with markdown style with image info like this
text:aaaaa
however, client user can only get the info as below. On our client side who use Microsoft Teams App, the image info could not be parsed normally, although image src is a public link.
I know by using attachment image can be send successfully, but what we need is to send a rich text which has ordered text and image as below
Could someone tell me how to find a solution?
I have tested the given markdown syntax in MS Team and it's rendering the correct output. Here through QnA maker I have verified & tested the flow instead of the API that you have given. The issue is not related to Teams APP and look like the markdown has one extra text in the url probably that creating the problem while posting attachment in the MS Teams through request body.
Your Markdown Syntax
Remove the extra "test" content from url.
text:aaaaa
Markdown Syntax
text:aaaaa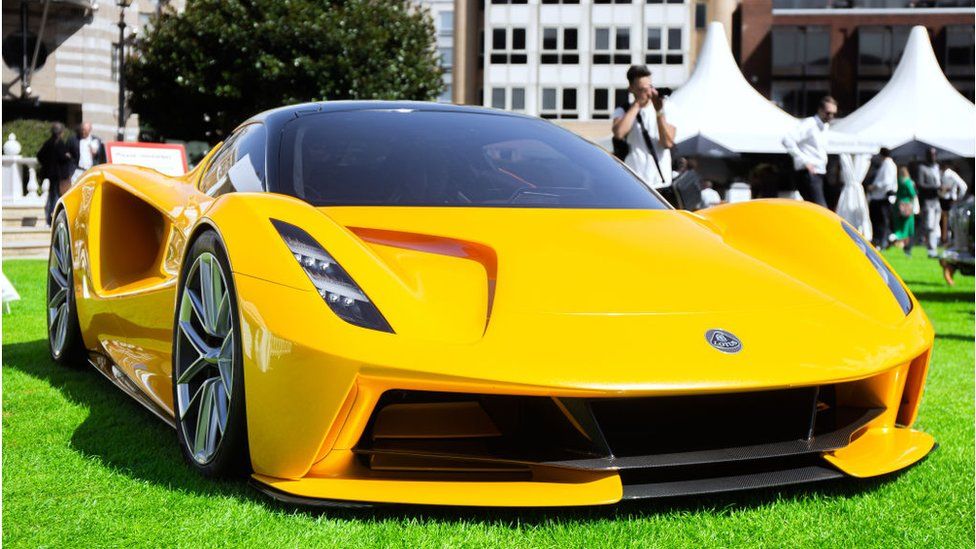
Output
Note: QnA maker will automatically remove this kind of extra content from the url during the build & test process.
This is possible using Language Generation file in your project and using Adaptive cards. You can find samples here https://adaptivecards.io/samples/
You can design your own adaptive card in your designer https://adaptivecards.io/designer/
and create a json file which you will have to place inside your project Template folder. (or respective folder directory that you have registered)
Once you are done creating and placing the files, follow the code to call the adaptive card
await dc.Context.SendActivityAsync(ActivityFactory.FromObject(_templates.Evaluate("yourlgintent"))).ConfigureAwait(false);
in your filename.lg file
# yourlgintent
[Activity
Attachments = ${ActivityAttachment(json(fromFile('yourfilename.json')), 'adaptiveCard')}
]
OR
You can directly call it in a method
# yourlgintent
[Activity
Attachments = ${ActivityAttachment(json(yourlgintentJson()), 'adaptiveCard')}
]
#yourlgintentJson
- ```
{
"$schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"type": "AdaptiveCard",
"version": "1.3",
"body": [
{
"type": "Container",
"items": [
{
"type": "TextBlock",
"wrap": true,
"text": "As your virtual assistant, I love answering your questions. Having said that, I am learning continuously to expand my knowledge.",
"height": "stretch"
}
],
"spacing": "Large"
}
]
}
And yes, If you are looking to get image from your QnA, then follow the below code
Inside your QnAMaker portal ,
Your Answer. [Image](https://URLTotheimage.com/Images/image.png)
This should give image within your answer.
Finally I found the sollution
https://github.com/microsoft/AdaptiveCards/issues/4121
I have implemented Docusign's embedded signing using the apex toolkit for docusign. I have stored my document to be signed in Documents object. I want to add the sign here tab to the document and then send it to docusign. ANy ideas on how can I achieve this?
Below is the code i've used to generate the envelope. I need to add the sign here tab to the envelope:
Id mySourceId = '0012v00002WathI';
Id myDocumentId = '0692v00000AF1yP';
dfsle.Envelope myEnvelope = dfsle.EnvelopeService.getEmptyEnvelope(
new dfsle.Entity(mySourceId)) // The initiating Salesforce entity.
.withDocuments(dfsle.DocumentService.getDocuments(
ContentVersion.getSObjectType(),
new Set<Id> {
myDocumentId
}))
.withRecipients(new List<dfsle.Recipient> {
dfsle.Recipient.newEmbeddedSigner(),
});
myEnvelope = dfsle.EnvelopeService.sendEnvelope(
myEnvelope, // The envelope to send
true); // Send now?
I may be wrong but from what I remember when working with the apex toolkit it does not allow you to add tags to documents through Apex. I'm pretty sure what you have there should work and when you initiate the embedded signing process you will get to choose where the sign tags go. You could set up a template for this document to decide where the tags go beforehand (which is useful if you send the same document often) for how to add documents with templates to envelopes see this page at step 3. The toolkit documentation isn't very clear so you may want to watch a demo to see how the whole process works like this one. Hope this helps.
First of all - all code on PHP and JS.
I took all user data (sub, base_uri, account_id ) with that documentation https://developers.docusign.com/esign-rest-api/code-examples/config-and-auth at my site using cURL
Now I need send pdf file for that I generate at my site with that access for user sign like I have understood I need that https://developers.docusign.com/esign-rest-api/code-examples/signing-from-your-app and Envelopes: create https://developers.docusign.com/esign-rest-api/reference/Envelopes/Envelopes/create#examples
I send POST request to https://demo.docusign.net/restapi/v2/accounts/{accountId}/envelopes with accountId=account_id from user data, also I send json with user data and doc data in base64 in, but there is nothing, so I don't understand:
How should I call "create" method with POST to https://demo.docusign.net/restapi/v2/accounts/{accountId}/envelopes ?
What Id should I use in {accountId}?
At what format should I send the document for the sign?
Where can I find that doc after create?
Can you show me code example or show documentation with normal examples of POST request for that method.
If you are using a demo environment, I believe your authentication url is https://demo.docusign.net/restapi/v2/login_information. For production accounts, since the subdomain can be different you can find the correct baseUrl using a GET request to https://www.docusign.net/restapi/v2/login_information?api_password=true using Postman. This gives you the right baseUrl as well as your authentication information.
Here is how you can create a template using a base64 which is a POST call to {{baseUrl}}/templates
{
"documents": [
{
"documentBase64": "<insert encoded base64 here",
"documentId": "1",
"name": "blank1.pdf"
}
],
"envelopeTemplateDefinition": {}
}
Also, if you need to generate more requests make sure you check out the Postman collection that contains different examples as Sebastian mentioned too:
DocuSign Postman Collection
I'm currently trying to build a private app which will allow me to create a form which customers can use to update info like name, email address, etc.
I know that I can access this information in my template through the customer object:
https://help.shopify.com/themes/liquid/objects/customer
I also believe that I can send http requests through the admin api which would allow me to update a given customer object:
https://help.shopify.com/api/reference/customer#update
This is an example PUT request from that page
PUT /admin/customers/#{id}.json
{
"customer": {
"id": 207119551,
"email": "changed#email.address.com",
"note": "Customer is a great guy"
}
}
I think that in order to use this api (or at least use it securely) I need to use a private app. I found the following npm package which I would use to create the private app:
https://www.npmjs.com/package/shopify-node-api
This is an example of a PUT request from that page (I think this can be modified for customers):
var put_data = {
"product": {
"body_html": "<strong>Updated!</strong>"
}
}
Shopify.put('/admin/products/1234567.json', put_data, function(err, data, headers){
console.log(data);
});
Does anyone have any experience doing this as I'm unsure about a few things.
Will this PUT request be called when the url is loaded? So if I have an
<a> tag with href="/admin/products/1234567.json the request would load?
If so, this seems quite useless with the customer ID hardcoded in. Can I pass in the customer ID of whoever is logged in and clicking the link and use that as the last part of the request url somehow? In addition to this would it be possible to grab the form data that the user enters to use as the value for "email" or "note?
You should check out this answer shopify app proxy: send customer data or only customer ID for some pointers, discussion and links.
tl/dr; Don't rely on only the logged in customer id or you'll be opening yourself up to easy hackery.
So bascially you update the customer with the PUT you outlined in your question.
To get the id securely you:
Create a form with the customer id and make sure you have a server generated hash of that customer id to thwart bots (that's the reference post)
You post the customer data to a an app via a proxy url
You update the customer via a PUT to a constructed url.
i'm using the Box View API to convert a PDF file to HTML, i'm using the /documents/{id}/content.{extension} section.
The response for this GET call is a .zip file, however i don't know how to retrive it and make downloadable.
Also note that i'm using node.js.
Thanks for your help
You can set your own webhook URL that will be called by Box when your document status changes (one POST on your webhook for "document.viewable", and one for "document.done" plus one "document.error" if an transformation error occured).
Just listen to the "document.done" status and download the assets then. Format that is posted to the webhook you have set looks like :
[{
"type": "document.done",
"data": {
"id": "4cca28f1159c4f368193d5014fabc16e"
},
"triggered_at": "2014-01-30T20:33:04.798Z"
}]
Beware of the docs and check the format programatically. Their API docs are often no quite correct and they post multiple webhooks at the time i'm writing (which is a bug i've reported).
For more info and Box View API docs