i'm using the Box View API to convert a PDF file to HTML, i'm using the /documents/{id}/content.{extension} section.
The response for this GET call is a .zip file, however i don't know how to retrive it and make downloadable.
Also note that i'm using node.js.
Thanks for your help
You can set your own webhook URL that will be called by Box when your document status changes (one POST on your webhook for "document.viewable", and one for "document.done" plus one "document.error" if an transformation error occured).
Just listen to the "document.done" status and download the assets then. Format that is posted to the webhook you have set looks like :
[{
"type": "document.done",
"data": {
"id": "4cca28f1159c4f368193d5014fabc16e"
},
"triggered_at": "2014-01-30T20:33:04.798Z"
}]
Beware of the docs and check the format programatically. Their API docs are often no quite correct and they post multiple webhooks at the time i'm writing (which is a bug i've reported).
For more info and Box View API docs
Related
I'm using the following api to successfully get file data:
https://acme.sharepoint.com/sites/my-site/_api/Web/Lists(guid'xxx')/files('yyy')
This is a docx file on which I've posted comments using the web console.
How can I fetch these comments using the rest api? I tried appending /comments to the url, but I'm getting the following 404 error:
{
"error": {
"code": "-1, Microsoft.SharePoint.Client.ResourceNotFoundException",
"message": {
"lang": "en-US",
"value": "Cannot find resource for the request Comments."
}
}
}
The Comments() endpoint currently exists only under the Items() endpoint and not under the Files() endpoint.
Basically, you can access the Comments() functionality only under the below endpoint:
GET https://{site_url}/_api/web/lists/GetByTitle({list_title})/items({item_id})/Comments
You can easily test the above in a PowerAutomate scenario with a Send Http Request to SharePoint actions.
In the below example I attempt to target the file in the document library:
On the other hand, if I attempt to target the file based on the List Item Id that it got in the document library I will get the below response:
As you can see from the above, I am also able to target a specific comment that I left.
Please take note of the below
The Comments() endpoint is not available for MS resources, meaning docx, excels and such files. It is only available for non-MS resource files like pdfs, txts and so on. I am not sure why this rule is in effect but, my best guess would be because there is a "commenting" functionality provided within a Word Document, for example.
You could find a bit more info about the above here.
I'm trying to watch for google drive changes, and write down history of changes in database, also I want to store the files (all versions).
For now I have set up webhook, so I get some info in request.header, but this info looks weird, only message number differs, resourceUri or pageToken in it are the same.
Request headers don't contain info about file or folder that was changed. No change id, no file id, no actors.
I want to know, how this method is helpful and what should I do to get real-time changes with information about files that were changed.
There are some steps to handle Drive file changes.
1. Choose the change type you want to track
It could be just changes within the file, changes in the file metadata, such as moving the file to another folder or to a shared drive. You might want to use the Reports API for that one.
2. Watch for changes
You can use the Changes API to detect what data has changed or has been removed. If you want detailed information about the changes, you can also check the Activity API
3. Get the notification
Set up a webhook when changes are detected on the file
POST https://www.googleapis.com/drive/v3/files/fileId/watch
Authorization: Bearer auth_token_for_current_user
Content-Type: application/json
{
"id": "01234567-89ab-cdef-0123456789ab", // Your channel ID.
"type": "web_hook",
"address": "https://example.com/notifications", // Your receiving URL.
...
"token": "target=myApp-myFilesChannelDest", // (Optional) Your channel token.
"expiration": 1426325213000 // (Optional) Your requested channel expiration time.
}
Sample code from https://developers.google.com/drive/api/v3/push
4. Export the new version of the file
After a new change is triggered, you can download the new file version using the export method
You can check a more detailed guide on https://developers.google.com/drive/api/v3/manage-revisions
Recently we are building a application which send message through Microsoft BotFramework API
We are using the API as below
https://learn.microsoft.com/en-us/azure/bot-service/rest-api/bot-framework-rest-connector-api-reference?view=azure-bot-service-4.0#send-to-conversation
The following API allow us to send text or attachments by passing activities as parameters.
https://learn.microsoft.com/en-us/azure/bot-service/rest-api/bot-framework-rest-connector-api-reference?view=azure-bot-service-4.0#activity-object
Everything works well, and on our client side, text and attachments can be received normally as below.
However, recently we have to send rich text which contains text and image info. For example, admin user want to send text and pasted image together to client user.
So we are considering sending text with markdown style with image info like this
text:aaaaa
however, client user can only get the info as below. On our client side who use Microsoft Teams App, the image info could not be parsed normally, although image src is a public link.
I know by using attachment image can be send successfully, but what we need is to send a rich text which has ordered text and image as below
Could someone tell me how to find a solution?
I have tested the given markdown syntax in MS Team and it's rendering the correct output. Here through QnA maker I have verified & tested the flow instead of the API that you have given. The issue is not related to Teams APP and look like the markdown has one extra text in the url probably that creating the problem while posting attachment in the MS Teams through request body.
Your Markdown Syntax
Remove the extra "test" content from url.
text:aaaaa
Markdown Syntax
text:aaaaa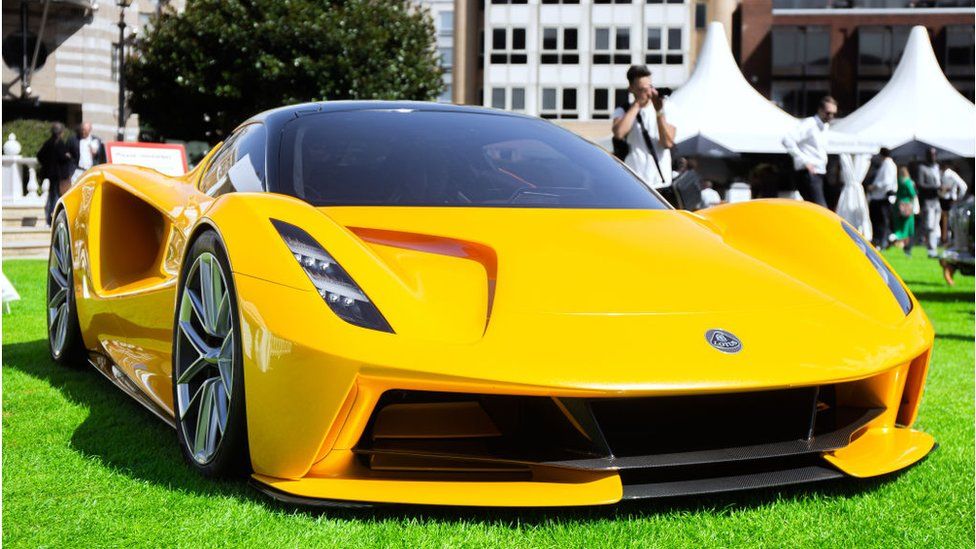
Output
Note: QnA maker will automatically remove this kind of extra content from the url during the build & test process.
This is possible using Language Generation file in your project and using Adaptive cards. You can find samples here https://adaptivecards.io/samples/
You can design your own adaptive card in your designer https://adaptivecards.io/designer/
and create a json file which you will have to place inside your project Template folder. (or respective folder directory that you have registered)
Once you are done creating and placing the files, follow the code to call the adaptive card
await dc.Context.SendActivityAsync(ActivityFactory.FromObject(_templates.Evaluate("yourlgintent"))).ConfigureAwait(false);
in your filename.lg file
# yourlgintent
[Activity
Attachments = ${ActivityAttachment(json(fromFile('yourfilename.json')), 'adaptiveCard')}
]
OR
You can directly call it in a method
# yourlgintent
[Activity
Attachments = ${ActivityAttachment(json(yourlgintentJson()), 'adaptiveCard')}
]
#yourlgintentJson
- ```
{
"$schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"type": "AdaptiveCard",
"version": "1.3",
"body": [
{
"type": "Container",
"items": [
{
"type": "TextBlock",
"wrap": true,
"text": "As your virtual assistant, I love answering your questions. Having said that, I am learning continuously to expand my knowledge.",
"height": "stretch"
}
],
"spacing": "Large"
}
]
}
And yes, If you are looking to get image from your QnA, then follow the below code
Inside your QnAMaker portal ,
Your Answer. [Image](https://URLTotheimage.com/Images/image.png)
This should give image within your answer.
Finally I found the sollution
https://github.com/microsoft/AdaptiveCards/issues/4121
I have list of users. When I click submit detail then
I want to create PDF file dynamically according to users detail and send with email to all users in nodejs.
This isn't really something that MailGun is designed to handle. You're responsible for creating the content and they're responsible for sending the content.
If you need to create a PDF dynamically, I'd recommend using Node or PHP to create your content as HTML/CSS and then using a library like html2pdf or a service like PDFShift to render your output in PDF form.
If you want to create a PDF dynamically i would suggest you to use pdf-creator-node
and for sending mails i would suggest you to use nodemailer
you can use this repo.
Check out Docamatic. It allows you to to create and send a PDF in a single API request.
{
"source": "https://my-site.com/invoice.html",
"email": {
"to": "accounts#my-customer.com",
"subject": "July Invoice"
}
}
I am using Docusign create envelop API using the endpoint https://au.docusign.net/restapi/v2/accounts/{AccountID}/envelopes. The API call works on most instance but occasionally I am receiving an error stating that the System was unable to convert this document to a PDF. I am submitting a docx type document which is failing randomly(say once in a day). On re submission the same document submission works without any issue.
In order to understand the problem I tried enabling logging on DocuSign login. Since the log can only keep upto 50 entries at any given time I am clearing the log to ensure I am ready to capture the failure when it happens.
Actual Error Message received:
{
"errorCode": "UNABLE_TO_CONVERT_DOCUMENT",
"message": "System was unable to convert this document to a PDF. Unable to convert Document(Document Name.docx) to a PDF. Error: UserId:{GUID} IPAddress:XX.XX.XXX.XXX Source:ApiRESTv2:Failed to convert FileType: docx"
}
Now I am trying to download the log file via DocuSign UI and I am receiving constant timeout issue while trying to do so. Does anyone know about any programmatic log file extract from DocuSign? Anyone done this previously?
Any pointers on the error resolution or help downloading the error log is much appreciated. Please help
The DocuSign platform by default accepts PDF documents so when sending through the API you simply need to include the document bytes for PDFs. However for any other file format you need to set the fileExtension property on the document object to the file type you are sending.
For example if using one of the open source DocuSign SDKs use the setFileExtension() setter method to set the extension:
document.setFileExtension("docx");
Or if you are calling the REST API directly (ie not using an SDK) then set the fileExtension property to "docx":
{
...
"fileExtension": "docx",
....
}
If you still receive the error after this then you I would start testing with a different document and confirm the document you are using not corrupt in any way and if properly formatted.