I'm working on an Azure Chatbot that will be connected to Facebook Messenger. The purpose of the bot is to look for the phrase of the day more or less. I currently have a url that returns the phrase of the day in plain text.
What I need the bot to do is for example:
User: "Hi, what is the phrase for today?"
The Bot will search the url and retrieve the plain text returned.
Bot: "The phrase for today is 'Don't Give Up!'"
I'm currently using QnA Maker for a knowledgebase, but it only works for static FAQs, not for pulling text from a website. Any help will be appreciated.
Thanks,
Axel
There's a couple of different ways you can go about this:
Programmatically Update Your QnA Knowledgebase
You'd need to create some kind of separate function (maybe using Azure Functions) outside of your bot that makes this API request every day.
curl -v -X PATCH "https://westus.api.cognitive.microsoft.com/qnamaker/v4.0/knowledgebases/{kbId}"
-H "Content-Type: application/json"
-H "Ocp-Apim-Subscription-Key: {subscription key}"
--data-ascii "{body}"
Body:
{
"add": {
"qnaList": [
{
"id": 0,
"answer": "You can change the default message if you use the QnAMakerDialog. See this for details: https://docs.botframework.com/en-us/azure-bot-service/templates/qnamaker/#navtitle",
"source": "Custom Editorial",
"questions": [
"How can I change the default message from QnA Maker?"
],
"metadata": []
},
[...]
Have the bot use an HTTP Request to get the Daily Phrase
Alternatively:
You would start by either intercepting messages that equal/contain:
"Hi, what is the phrase for today?"
Or, if you want the user's question to be a little more flexible, you could use LUIS to parse user input and return an intent.
Once you code that into your bot, just have the bot make a regular HTTP request to your website to the phrase, then send that to the user.
I can provide some code examples, if needed, but please provide a code sample of where in your bot you want this to occur. The URL for your phrase may also be helpful.
Related
Recently we are building a application which send message through Microsoft BotFramework API
We are using the API as below
https://learn.microsoft.com/en-us/azure/bot-service/rest-api/bot-framework-rest-connector-api-reference?view=azure-bot-service-4.0#send-to-conversation
The following API allow us to send text or attachments by passing activities as parameters.
https://learn.microsoft.com/en-us/azure/bot-service/rest-api/bot-framework-rest-connector-api-reference?view=azure-bot-service-4.0#activity-object
Everything works well, and on our client side, text and attachments can be received normally as below.
However, recently we have to send rich text which contains text and image info. For example, admin user want to send text and pasted image together to client user.
So we are considering sending text with markdown style with image info like this
text:aaaaa
however, client user can only get the info as below. On our client side who use Microsoft Teams App, the image info could not be parsed normally, although image src is a public link.
I know by using attachment image can be send successfully, but what we need is to send a rich text which has ordered text and image as below
Could someone tell me how to find a solution?
I have tested the given markdown syntax in MS Team and it's rendering the correct output. Here through QnA maker I have verified & tested the flow instead of the API that you have given. The issue is not related to Teams APP and look like the markdown has one extra text in the url probably that creating the problem while posting attachment in the MS Teams through request body.
Your Markdown Syntax
Remove the extra "test" content from url.
text:aaaaa
Markdown Syntax
text:aaaaa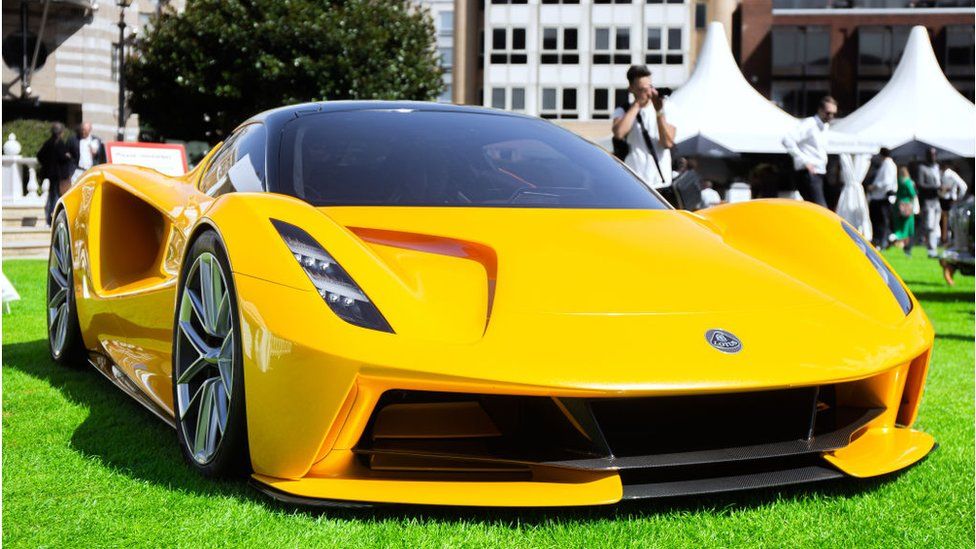
Output
Note: QnA maker will automatically remove this kind of extra content from the url during the build & test process.
This is possible using Language Generation file in your project and using Adaptive cards. You can find samples here https://adaptivecards.io/samples/
You can design your own adaptive card in your designer https://adaptivecards.io/designer/
and create a json file which you will have to place inside your project Template folder. (or respective folder directory that you have registered)
Once you are done creating and placing the files, follow the code to call the adaptive card
await dc.Context.SendActivityAsync(ActivityFactory.FromObject(_templates.Evaluate("yourlgintent"))).ConfigureAwait(false);
in your filename.lg file
# yourlgintent
[Activity
Attachments = ${ActivityAttachment(json(fromFile('yourfilename.json')), 'adaptiveCard')}
]
OR
You can directly call it in a method
# yourlgintent
[Activity
Attachments = ${ActivityAttachment(json(yourlgintentJson()), 'adaptiveCard')}
]
#yourlgintentJson
- ```
{
"$schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"type": "AdaptiveCard",
"version": "1.3",
"body": [
{
"type": "Container",
"items": [
{
"type": "TextBlock",
"wrap": true,
"text": "As your virtual assistant, I love answering your questions. Having said that, I am learning continuously to expand my knowledge.",
"height": "stretch"
}
],
"spacing": "Large"
}
]
}
And yes, If you are looking to get image from your QnA, then follow the below code
Inside your QnAMaker portal ,
Your Answer. [Image](https://URLTotheimage.com/Images/image.png)
This should give image within your answer.
Finally I found the sollution
https://github.com/microsoft/AdaptiveCards/issues/4121
I am creating a simple bot using Azure LUIS and this is my first one. I made some decent progress after doing some research and also now integrated with Slack as channel to test it.
The bot functionality is working fine, but I am looking to identify the user. So that I can personalize the bot conversation and also to pull the user specific information from his profile table.
Is there anyway, that I can get a UID or any reference ID of the slack user and so I can store that in my user table along with user profile?
So next time, when the user greets the bot, the bot can say "Hello, John." instead of justing say "Hello."
Thanks!
Yes. You can use the channelData object to get the ApiToken, and user values. For example, in C#, you could use turnContext.Activity.ChannelData to get those values in JSON:
{{
"SlackMessage": {
"token": "............",
"team_id": "<TEAM ID>",
"event": {
"type": "message",
"text": "thanks",
"user": "<USER WHO MESSAGED>",
"channel": "............",
"channel_type": "channel"
},
"type": "event_callback",
"event_id": ""............",
"event_time": 1553119134,
"authed_users": [
"............",
"<USER WHO MESSAGED>"
]
},
"ApiToken": "<ACTUAL TOKEN HERE>"
}}
Then, using those two pieces of information, you can then retrieve info from Slack.
https://slack.com/api/users.info?token=<ACTUAL TOKEN HERE>&user=<USER WHO MESSAGED>&pretty=1
And get a response that has the info you need:
{
"ok": true,
"user": {
"id": "<USER WHO MESSAGED>",
"team_id": "<TEAM ID>",
"real_name": "Dana V",
Ideally, you would would probably want to have bot user state setup and check that first, then if not there, then make the API call to Slack, then store in state. Therefore further requests don't need to go to Slack, but will just pull from the state store.
Basically, you could/should do this in the onTurn event. First, create your user state storage such as here.
Then you could check for that value and write to it if not populated. This example on simple prompts, might be helpful. You won't need to prompt for your user's name, as this example does, but does read/write username from state. You could still use dialogs, but you won't need them for the name prompting as you are doing that dynamically.
You can see here where username is being set and here where it is being retrieved. In this case, it is in the dialogs, but again; you would/could just do in the turn context (using logic to get and if not there, set).
I found the solution by priting the whole session object, which is having all the required informaiton. This could be same as mentioned by Dana above, but after debugging, this follwing made simple without making any changes.
var slackID = session.message.address.user.id
With above, I am able to identify the user.
Thanks.
I've created a chatbot using Dialogflow and integrated it with Telegram, Facebook Messenger and Web.
The response for Dialogflow is created via Fulfillment written in Python.
In Telegram and Facebook Messenger I am getting replies as expected for each message from user.
But now when I am trying integration for Web, I've noticed that the replies from chatbot does not show multiple reply messages/lines and Quick Replies.
Below is screenshot when user say 'Hi' to the chat bot in Telegram, Facebook Messenger, Web and Dialogflow console respectively. Why is this happening and how can I fix this?
Below is the Fulfillment response JSON:
{
"fulfillmentMessages": [
{
"text": {
"text": [
"Greetings from Tulsi Village!!!",
"My name is Appu. I am your virtual assistant.",
"How can I help you?"
]
}
},
{
"quickReplies": {
"quickReplies": [
"Book",
"Rooms",
"Contact",
"Other"
]
}
}
]
}
The rendering of response depends on the client you are using.
Telegram, Facebook Messenger, are able to render the quick-replies and multi-line replies.
But the web-demo which you are using does not support these, hence you are not able to see quick-replies and multi-line replies.
If you want to integrate it with a website, you need to design it in such a way that it can render the json response and show it correctly. Web-demo is not designed to render these.
Microsoft Bot Framework messages with buttons in Facebook Messenger
My question relates to the question linked aboved. I am writing a bot using node.js that does not use the bot builder sdk. I manually returning a compatible response for the ms bot connector service. This is working fine for a text response, but I wish to return more complicated responses, e.g the buttons/carousel you can return with messenger. Based on the question I linked above, I guessed the format and added the below:
response.attachments = [ { "Title": "Choose One: ", "Actions": [{ "Title": "Postback!", "Message": "Postback from button" }, { "Title": "Postback2!", "Message": "Postback2 from button" }] } ];
The top level title seems to do nothing but the actions render as postback type buttons correctly (they send the Message as the postback content). With messenger you also have the option to return url based buttons, and image urls.
As far as I can tell there is zero documentation on returning attachments using the node bot builder sdk. If there were I'd just write the bot with the sdk in order to obtain the response format.
So my question is, does anyone know how to correctly return both postback and url based buttons to the bot connnector service, including accompanying images, with or without the bot builder sdk?
Update 05/05/2016
So I found the link below and you can see a definition of the attachments property:
http://docs.botframework.com/sdkreference/nodejs/interfaces/_botbuilder_d_.imessage.html
If you follow it to the IAttachment specification, it makes me wonder how/why my code above works at all? As a test of that format I wrote in the following:
var att = {};
att.content = "I am content";
att.contentType = "text/plain";
att.contentUrl = "http://www.google.com";
att.fallbackText = "I am fallback text";
att.text = "I am text";
att.thumbnailUrl = "https://pbs.twimg.com/profile_images/638751551457103872/KN-NzuRl.png";
att.title ="I am title";
att.titleLink = "http://yahoo.com";
Now in slack I get a fairly nice output from this:
However in messenger I get "Service Error:Value cannot be null. Parameter name: source"
I found the info I needed. Not sure if it hadn't been published at the time or whether I was just hunting in the bot builder docs, but it's all detailed fairly well below.
http://docs.botframework.com/connector/message-actions/#navtitle
You have to tweak your message a little for certain integrations, e.g Skype doesn't really seem to support attachments.
Does anyone know of an service / api that lets you send a group message? I've been searching through Twilio's docs - seems like you can only send a message to one person.
Ideal use case:
[API Number] ----Sends Text----> (Friend 1 + Friend 2 + Friend 3)
And the result is a group message with 4 people in it - the three friends and the API.
d7networks.com is doing it with their RESTful API
========================================================
curl -X POST -H 'Authorization: Basic xxxxxxx==' -d '{
"messages": [
{
"to": [
"777771",
"777772",
"777773"
],
"content": "Same content goes to 3 numbers",
"from": "TEST"
}
]
}' http://sms.d7networks.com:8080/secure/sendbatch
You can't do it exactly as you describe but you can build something that creates a group SMS where everyone is texting the same #. This blog post shows how to build a group messaging app like this with Meteor, MongoDB and Twilio.
As far as I can tell http://www.bandwidth.com is the only sms api that supports group texting. You can see it in action at https://bandwidth.wistia.com/medias/ymd7q9utm0#. Unfortunately their trial doesn't have access to this feature (the trial uses api v1 and this is a feature of api v2) and there's no easy way to sign up for an account.